While the creation of velocity widgets is quick and easy, you’ll might come across use cases that can only be tackled with the creation of a Java based widgets. This is mostly the case as soon as you want to create an interactive widget. In this post I want to go trough the basics of Java widget development for Polarion.
Java can be used to create workflow functions & conditions, form extensions, servlets and widgets. But instead of using Java, I personally used Javascript and Velocity as long as possible. But recently I came across use cases which need me to implement it by using Java. And there are other advantages like a thorough way of debugging.
We’ll go trough the following topics:
- Preparing Eclipse Workspace
- The RichPageWidget class
- Methods to implement
- Export as deployable Widget
- Troubleshooting
Preparing Eclipse Workspace
In order for us to create a Java widget, we have to set up our IDE first. Although e.g. IntelliJ should also be possible and might be more user friendly, I use Eclipse as this is what Siemens uses for the development and also provides documentation for.
- Install Polarion (locally, you can use the 30-day trial for it)
- Set up eclipse workspace according to chapter 4.3: Polarion SDK Documentation
- Import the example widget from your [Polarion install directory]/polarion/SDK/examples or download it here: Example Widget
- Shutdown your Polarion Server (keep Apache and Postgre running) and start it via your Eclipse by pressing the run or debug symbol. (Maybe turn off the Polarion autostart in the services settings of Windows, set the service sype to manual)
This will start-up your Polarion server and all widget-projects, that were opened in your Eclipse workspace. These widgets will be loaded and available in Polarion. (The example widget is called „Work Record Report Widget“)
The RichPageWidget class
What makes a project in Eclipse a widget in Polarion? Answer: There has to be one class extending the RichPageWidget class of Polarion.
public class WorkRecordReportWidget extends RichPageWidget {}
This class contains a bunch of (abstract) methods that have to be implemented/overriden:
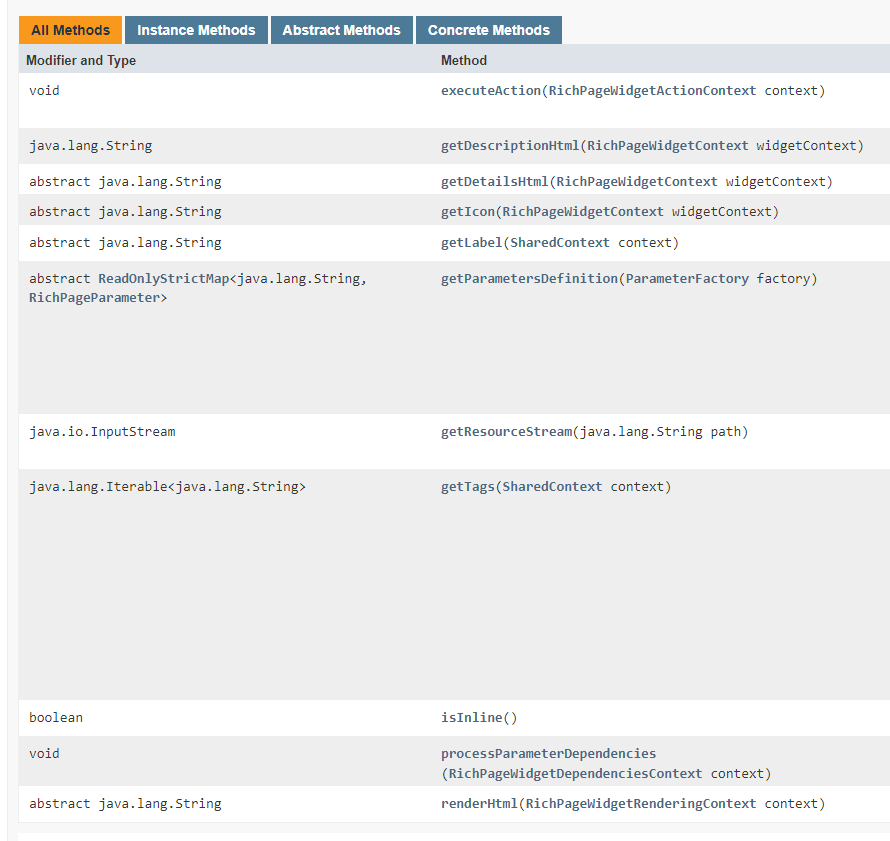
Methods to implement
You have to implement all methods that don’t have an implementation by the superclass RichPageWidget. You can identify them at the modifier „abstract“ in the column „Modifier and Type“.
There are the basic methods getLabel(), getDescription() and getIcon(), that allow you to set the title, description and icon of the widget.
To set the name of your widget you would override the getLabel() function and return the name as String.
@Override @NotNull public String getLabel(@NotNull final SharedContext context) { return "Work Record Report Widget"; }
This will result in the name being shown below the icon in the widget sidebar:
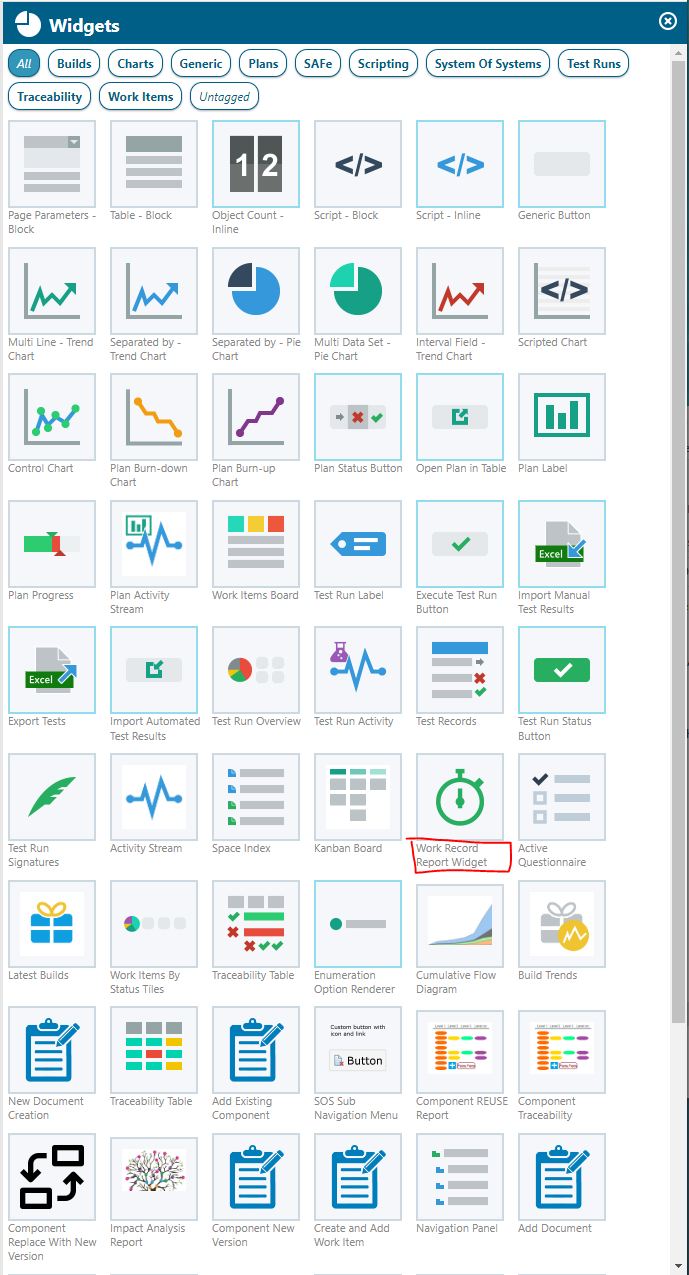
The method getParametersDefinition() is used to define the widget parameters. Therefore you have to create a map<String, RichPageParameter>. The String is just the name of the parameter and the parameter itself must be created with the ParameterFactory, which will be provided as parameter from Polarion. To add a simple String widget parameter the code could look like this:
StrictMap<String, RichPageParameter> parameters = new StrictMapImpl<String, RichPageParameter>(); // Factory is instance of ParameterFactory and provided by Polarion as parameter for the method parameters.put("parameterId", factory.string("Parameter Label").value("defaultValue").build()); return parameters;
And most importantly we have to implement the renderHtml() method, which will be the main method of the widget. It’s called when someone opens the report page, where this widget is inserted. So this is our starting point. As return value we have to provide the html and javascript as string.
Please be aware, that multiple instances of a widget can be placed inside of a report page, thereforen this applies:
Rich Page Widget is a visual component which can be added by the user to the Rich Page and which shows some data based on user defined parameters – see
Polarion API (RichPageWidget)getParametersDefinition(ParameterFactory)
Implementations should be state-less and not assume that only one instance is created in runtime.
Export as deployable Widget
After your development you would like to ship the widget so that it can be deployed an any Polarion by putting it into the [Install]/polarion/extensions folder. It is explained in chapter 4.4.: Polarion SDK. Basically you have to ensure your build.properties is set up correctly and then File>Export>Deployable Plugins and Fragments. It will create your .jar.
To be deployable the extension has to follow a certain folder structure. E.g.: …\extensions\com.polarion.alm.velocityform\eclipse\plugins\com.polarion.alm.velocityform_1.0.4\formExtension.jar & META-INF“
Troubleshooting
For general troubleshooting of the „widget development set up“, I recommend looking at existing widgets. You can get others on the extension portal with sources (e.g. Active Questionaire).
If something during the deployment as extension is not working, you should check the Polarion log for your widget. Polarion lists all widget that are loaded during the start-up in the logs and if there is something wrong with them.
You should also come back and write a comment about how you fixed it 😉
Packages can’t be resolved
- Check your workspace preparation
- Check if Manifest.mf is correctly configured
Can’t start Polarion via Eclipse
- Deactivate all linux/windows (depending on your system) specific plugins for the target platform.
- Check target platform configuration
- Select correct target platform, if you have multiple
Deployed Extension is not available
- Check that you deleted the „data/workspace/.config“ and restarted the Polarion service. The .config file cashes extensions.
- Ensure that you created the correct folder structure as explained in the chapter „Export as deployable Widget“